April 2012
Monthly Archive
April 30, 2012
Posted by Andrei Alexandrescu under
General
[3] Comments
Writing code that is resilient upon errors (API failures, exceptions, invalid memory access, and more) has always been a pain point in all languages. This being still largely an unsolved (and actually rather loosely-defined) problem, C++11 makes no claim of having solved it. However, C++11 is a more expressive language, and as always more expressive features can be put to good use toward devising better error-safe idioms and libraries.
This talk is a thorough visit through error resilience and how to achieve it in C++11. After a working definition, we go through a number of approaches and techniques, starting from the simplest and going all the way to file systems, storage with different performance and error profiles (think HDD vs. RAID vs. Flash vs. NAS), and more. As always, scaling up from in-process to inter-process to cross-machine to cross-datacenter entails different notions of correctness and resilience and different ways of achieving such.
To quote a classic, “one more thing”! An old acquaintance—ScopeGuard—will be present, with the note that ScopeGuard11 is much better (and much faster) than its former self.
April 30, 2012
Posted by Herb Sutter under
General
[2] Comments
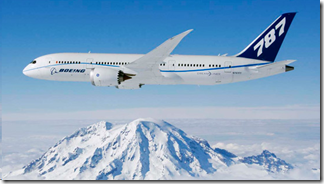
C++11 is kind of like “C++ Dreamliner.” It’s built with world-class modern materials. It took a couple more years to finish than originally expected. But now it’s starting to roll out worldwide and flying more safely and efficiently than ever. (European readers may equally consider it “C++ 380.”)
I write and teach, speaking about C++11 in particular:
“Modern C++ is clean, safe, and fast – modern C++ code is as readable and as type- and memory-safe as code written in any other modern language [*], and it has always been the king of ‘fast’ and is now faster than ever with new features like move semantics.” – Herb Sutter
Bjarne Stroustrup made me add, at the end of the “clean and safe” part:
“[*] When used in a modern style.” – Bjarne Stroustrup
Bjarne and I claim this to be true. Others have disputed various parts of this statement – whether C++11 really has a usable safe subset, whether C++ is really necessarily faster than code in other languages, and other questions. What’s the truth?
These answers matter. Type safety and memory safety are important not only for writing reliable code that will fail or be abused less often, but also for improving developer productivity so that developers can spend less time on overhead “taxes” like checking for unsafe casts or buffer overruns. And the claim that C++ really is the king of “fast” has been consistently challenged for most of its history.
In this panel, Scott, Andrei, and I will weigh in with discussion – and data – on these important and current questions.
April 16, 2012
Posted by scottmeyers under
General
[21] Comments
In recent talks (e.g., at Going Native in February and at Lang.Next earlier this month), Herb has offered guidelines for proper usage of C++11. He made it look so easy, I said to myself, “Heck, I could do that.” Besides, people have been pestering me about a new edition of Effective C++, so I figured I’d sit down and try to come up with a few prospective guidelines. “How hard can it be?,” I asked myself.
Easier than expected, actually. In about a half hour, I’d jotted down some 25 candidate guidelines. That’s more than enough for a meaty C&B talk, so I plan to give one. What will be in the talk? I don’t know yet, because what I have are prospective guidelines, and they will evolve as I work on them and as I learn more about C++11 and its effective application. (My knowledge continues to change almost daily.) What follows is a snapshot of the list of candidate guidelines I have right now. The ordering is random, and the wording is tentative, so don’t read too much into them. There are far more here than can be covered in single talk, so there’s no way I’ll cover all of these at C&B. My plan is to focus on the ones I think include material that is particularly important or particularly surprising (e.g., counterintuitive). If you see a topic you’d like to hear about, please post a comment to that effect. If you don’t see a topic you think I should address, please post about that. If you see a guideline you think is bad advice, posting a comment is again the thing to do. If you know of a good guideline you don’t see listed, post, post, post!
Scott
-
Prefer auto to Explicit Type Declarations
-
Distinguish () and {} When Creating Objects
-
Remember that auto + { expr } == std::initializer_list
-
Prefer non-member begin/end to member versions
-
Declare std::thread Members Last in Classes
-
Be Wary of Default Capture Modes in Lambdas Escaping Member Functions
-
Prefer Emplacement to Insertion
-
Pass std::launch::async if Asynchronicity is Essential
-
Minimize use of Weak Atomics
-
Distinguish Rvalue References from Universal References
-
Assume that move operations are neither present nor cheap
-
Prefer Lambdas over Binders
-
Prefer Lambdas over Variadic Arguments to Threading Functions
-
Be Wary of Oversubscription
-
Apply std::forward when Passing Universal References
-
Prefer std::array to Built-in Arrays
-
Use std::make_shared Whenever Possible
-
Prefer Pass-by-Reference-to-const to Pass-by-Value for std::shared_ptrs
-
Pass by Value if You’ll Copy Your Parameter
-
Reserve noexcept for Functions with Wide Interfaces
-
For Copyable Types, View Move as an Optimization of Copy
-
Prefer enum classes to enums
-
Prefer nullptr to NULL and 0
-
Distinguish among std::enable_if, static_assert, and =delete
April 8, 2012
Posted by scottmeyers under
General
[4] Comments
If you’re reading this blog, it can be taken for granted that you think C++ is a commercially important programming language. Today I came across Vincent Lextrait’s Programming Language Beacon, which summarizes the primary programming language used in nearly 100 major software products or utilities. I was not surprised that C++ was well-represented; I was astonished at how well-represented it was!
Being an “anybody can publish anything on the Internet, so who knows if it’s true?” kind of guy, I did some cursory Googling for comments to the effect that Vincent Lextrait has no idea what he’s talking about. I found none. So I pinged Andrei and Herb to see if they knew about this table or could offer comments on its accuracy. They had not seen it, either, but when they reviewed the data in the table, they found it accurate for all the products they were familiar with.
We believe that the table is legitimate, and if you have not seen it, we encourage you to take a look. As I said, as a reader of this blog, you’re already a C++ believer, but this table may make you an even bigger believer, and it may also help you explain to others at least part of the reason why you are. Personally, my favorite part is where the table shows that Java’s JVM and .NET’s CLR are both written in C++, but that’s far from the only area where C++ trumps the competition.
At C++ and Beyond in August, Andrei and Herb and I will do our best to equip you with information that will help you add to C++’s lead on a list that it already dominates.
Scott
PS - If you’re interested in other lists that demonstrate C++’s widespread commercial utilization, take a look at Bjarne’s C++ Applications page.
April 2, 2012
Posted by scottmeyers under
General
[11] Comments
You can’t do a better job if you don’t change what you’re doing, but change is hard. It’s especially hard when what needs to change is your colleagues’ approach to software development. Moving your team forward often requires persuading your peers to change their behavior, sometimes to do something they’re not doing, other times to stop doing something they’ve become accustomed to. Whether the issue is to embrace or avoid C++ language features, to adopt new development tools or abandon old ones, to increase use of or scale back on overuse of design patterns, to adhere to coding standards, or any of the plethora of other matters that affect software creation, moving things forward typically requires getting your colleagues to buy into the change you’re proposing. But how can you do that?
Like you, Herb and Andrei and I have co-workers we sometimes need to nudge in one direction or another, and as authors, trainers, and consultants, we often find ourselves needing to convince diverse sets of people we barely know to modify what they do. In this panel session, the three of us share how we go about doing this, and, because specific examples are worth a thousand generalities, we take your questions, too. In fact, we encourage you to post your “I just can’t get my colleagues to see the light!” stories, questions, and advice as comments on this blog post.
Scott